How to use Sentry with your Remix apps
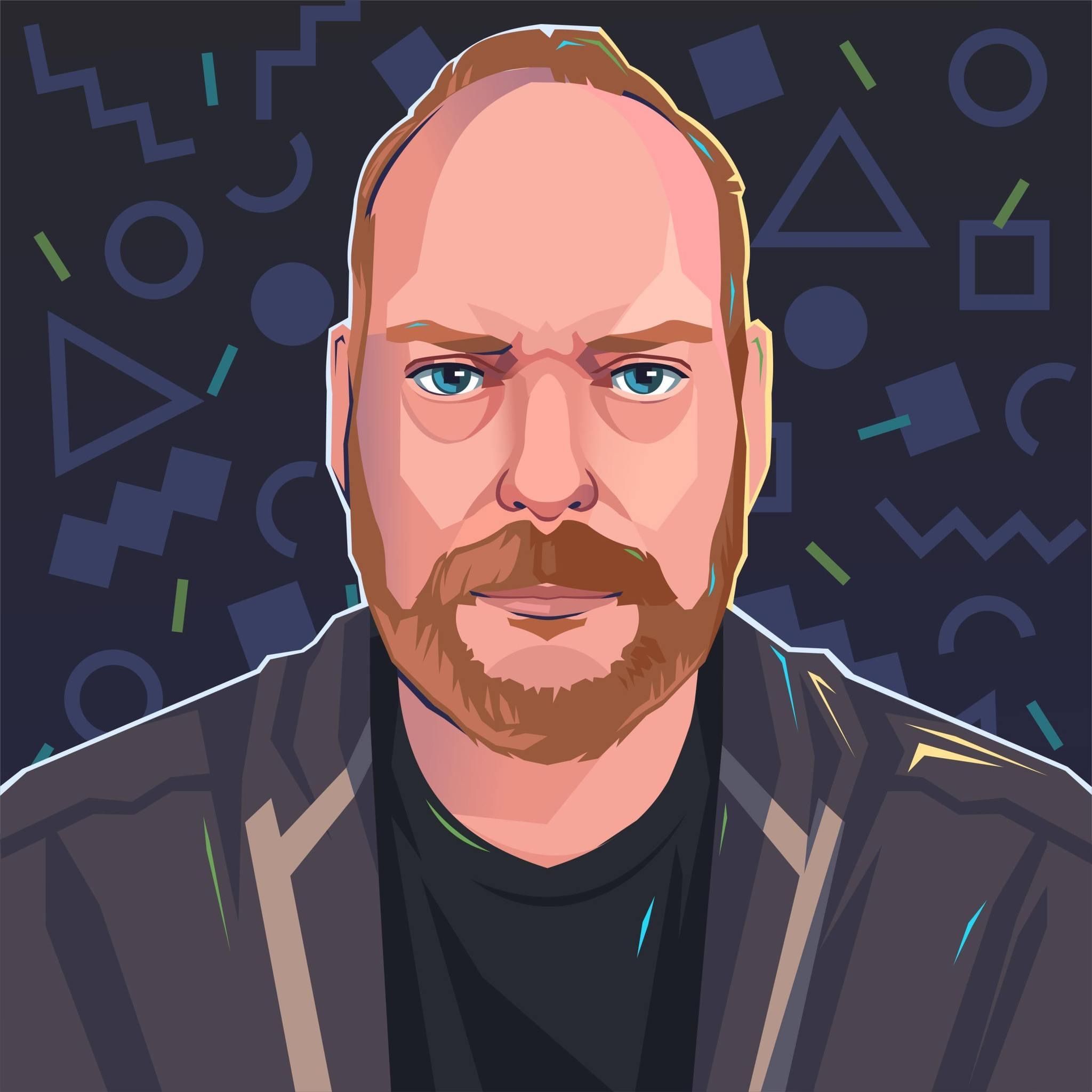
Sentry is great for error monitoring, and flexible.
This post will show how to add Sentry to your remix apps so it's set up for error, performance, and replay monitoring.
SaaS vs Self-Hosted
Sentry offers both a SaaS solution and self-hosted solution.
You can also use Glitchtip which has full support for the Senty API.
Signup
You can sign up for Sentry and create a Remix project from visting this url and filling out the signup form.
Onboarding
Once you see the onboarding page which has the DSN, copy that somewhere (this
becomes SENTRY_DSN
).
Install the Sentry SDK
To start, we'll have to install the @sentry/remix
package:
pnpm add @sentry/remix
Sentry's remix package works well for our needs so we'll use that.
You'll also need to add an environment variable called SENTRY_DSN
which is the client DSN sentry gave you when you created your project.
SENTRY_DSN=<your_dsn>
Set up monitoring files
We need to create two files, one for monitoring client side, and one for monitoring server side.
First, create a file called app/utils/monitoring.client.tsx
for client-side monitoring:
import { useLocation, useMatches } from '@remix-run/react'
import * as Sentry from '@sentry/remix'
import { useEffect } from 'react'
export function init() {
Sentry.init({
dsn: process.env.SENTRY_DSN,
integrations: [
new Sentry.BrowserTracing({
routingInstrumentation: Sentry.remixRouterInstrumentation(
useEffect,
useLocation,
useMatches,
),
}),
new Sentry.Replay(),
],
tracesSampleRate: 1.0,
replaysSessionSampleRate: 0.1,
replaysOnErrorSampleRate: 1.0,
})
}
Second, create a file called app/utils/monitoring.server.ts
for the server side monitoring:
import * as Sentry from '@sentry/remix'
export function init() {
Sentry.init({
dsn: process.env.SENTRY_DSN,
tracesSampleRate: 1,
})
}
Add monitoring to our entry files
We'll add our monitoring files to the appropriate entry files, so first let's edit entry.client.tsx
:
import { RemixBrowser } from '@remix-run/react'
import { startTransition } from 'react'
import { hydrateRoot } from 'react-dom/client'
if (process.env.NODE_ENV === 'production' && process.env.SENTRY_DSN) {
import('~/utils/monitoring.client').then(({ init }) => init())
}
startTransition(() => {
hydrateRoot(document, <RemixBrowser />)
})
This will load our monitoring.client
file if we are in production mode and the SENTRY_DSN
environment variable has been configured.
Next, let's edit our entry.server.tsx
file:
import { PassThrough } from "node:stream";
import type { EntryContext } from "@remix-run/node";
import { Response } from "@remix-run/node";
import { RemixServer } from "@remix-run/react";
import isbot from "isbot";
import { renderToPipeableStream } from "react-dom/server";
const ABORT_DELAY = 5_000;
if (process.env.NODE_ENV === 'production' && process.env.SENTRY_DSN) {
import('~/utils/monitoring.server').then(({ init }) => init())
}
export default function handleRequest(
request: Request,
responseStatusCode: number,
responseHeaders: Headers,
remixContext: EntryContext
) {
return isbot(request.headers.get("user-agent"))
? handleBotRequest(
request,
responseStatusCode,
responseHeaders,
remixContext
)
: handleBrowserRequest(
request,
responseStatusCode,
responseHeaders,
remixContext
);
}
function handleBotRequest(
request: Request,
responseStatusCode: number,
responseHeaders: Headers,
remixContext: EntryContext
) {
return new Promise((resolve, reject) => {
const { pipe, abort } = renderToPipeableStream(
<RemixServer
context={remixContext}
url={request.url}
abortDelay={ABORT_DELAY}
/>,
{
onAllReady() {
const body = new PassThrough();
responseHeaders.set("Content-Type", "text/html");
resolve(
new Response(body, {
headers: responseHeaders,
status: responseStatusCode,
})
);
pipe(body);
},
onShellError(error: unknown) {
reject(error);
},
onError(error: unknown) {
responseStatusCode = 500;
console.error(error);
},
}
);
setTimeout(abort, ABORT_DELAY);
});
}
function handleBrowserRequest(
request: Request,
responseStatusCode: number,
responseHeaders: Headers,
remixContext: EntryContext
) {
return new Promise((resolve, reject) => {
const { pipe, abort } = renderToPipeableStream(
<RemixServer
context={remixContext}
url={request.url}
abortDelay={ABORT_DELAY}
/>,
{
onShellReady() {
const body = new PassThrough();
responseHeaders.set("Content-Type", "text/html");
resolve(
new Response(body, {
headers: responseHeaders,
status: responseStatusCode,
})
);
pipe(body);
},
onShellError(error: unknown) {
reject(error);
},
onError(error: unknown) {
console.error(error);
responseStatusCode = 500;
},
}
);
setTimeout(abort, ABORT_DELAY);
});
}
Note
💡FYI, I'm just using the entry.server and entry.client files from the Remix Indie Stack in this example. You may have other modifications in your entry files but the only ones that matter here are the sentry imports.
That's it, we've added sentry monitoring to both the server and client side of our app and now we'll start getting reports showing up in Sentry as errors happen.