Creating post previews in Strapi with Remix
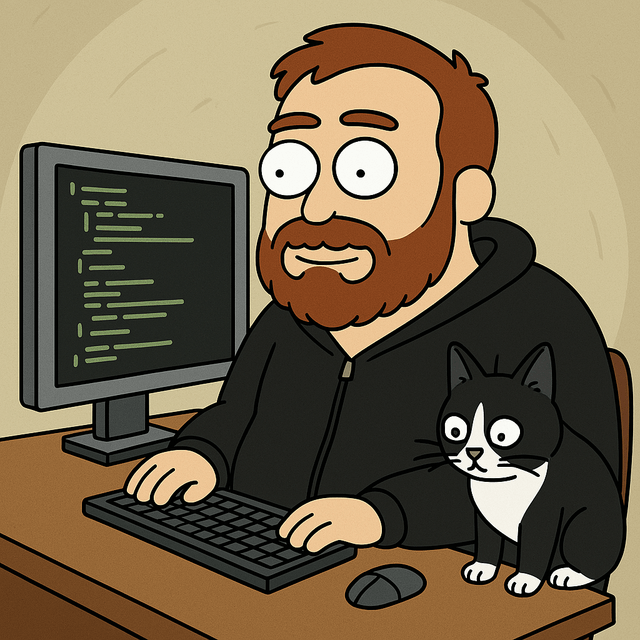
Being able to preview a new post is important, and when headless, it's even more important.
This little script sits at app/routes/blog/preview.tsx
(You can move it anywhere you want of course) and we'll call it with some query parameters such as post
for the post ID and secret
which will be an env var called PREVIEW_SECRET
:
import type { LoaderFunction } from "@remix-run/node";
import { json } from "@remix-run/node";
import { useLoaderData } from "@remix-run/react";
import { marked } from "marked";
import * as React from "react";
type Post = {
title: string;
article: string;
createdAt: string;
updatedAt: string;
publishedAt: string;
};
type PostData = { id: string; attributes: Post }[];
type PostResponse = {
data: PostData;
meta: {
pagination: {
page: number;
pageSize: number;
pageCount: number;
total: number;
};
};
};
export const loader: LoaderFunction = async ({request}) => {
const url = new URL(request.url);
const id = url.searchParams.get("post") || 1;
const secret = url.searchParams.get("secret") || 100;
if( !id ) {
throw json({ errors: [{
id: "post is invalid" ,
secret: null,
}] }, 400);
}
if( secret !== process.env.PREVIEW_SECRET ){
throw json({ errors: [{
id: null,
secret: "secret is invalid",
}] }, 403);
}
// This is where Remix integrates with Strapi
const response = await fetch(`http://localhost:1337/api/posts/${id}?publicationState=preview`);
const postResponse = (await response.json()) as PostResponse;
const post = postResponse.data;
return json(
{
...post,
attributes: {
...post.attributes,
article: marked(post.attributes.article),
},
}
};
const Posts: React.FC = () => {
const post = useLoaderData<PostData>();
const { title, article, createdAt } = post.attributes;
const date = new Date(createdAt).toLocaleString("en-US", {
weekday: "long",
year: "numeric",
month: "long",
day: "numeric",
});
return (
<article key={post.id}>
<h1>{title}</h1>
<time dateTime={createdAt}>{date}</time>
{/* Reminder that this can in fact be dangerous
https://reactjs.org/docs/dom-elements.html#dangerouslysetinnerhtml */}
<div dangerouslySetInnerHTML={{ __html: article }} />
</article>
);
};
export default Posts;
The big part of this page is that is uses publicationState=preview
when calling Strapi, this means you can return posts that are not yet live
in the Strapi database and lets you preview it. Obviously this makes the secret important but that's also up to you.
This is pretty light overall, since you're probably going to need to customize fields, etc for your own Strapi setup, as well as how it looks but I wanted to share this as a starting point.